| Due date: 2/11 @ noon
Objective: to create a test suite; to implement a testing
framework if needed
The following table displays 11 graphs and their construction:
image of graph | construction of graph |
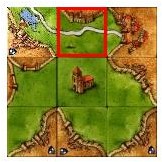 |
abbey1.ss
add tile "11" at (-1,0) with orientation 180
add tile "14" at (-1,1) with orientation 270
add tile "9" at (-1,2) with orientation 90
add tile "9" at (0,2) with orientation 180
add tile "9" at (1,2) with orientation 90
add tile "8" at (1,1) with orientation 0
add tile "6" at (1,0) with orientation 90
add tile "1" at (0,1) with orientation 0
|
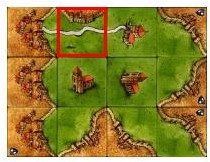 |
abbey2.ss
add tile "1" at (1,1) with orientation 90
add tile "9" at (2,0) with orientation 0
add tile "9" at (2,1) with orientation 90
add tile "9" at (2,2) with orientation 0
add tile "8" at (1,2) with orientation 180
add tile "8" at (0,2) with orientation 90
add tile "6" at (-1,0) with orientation 270
add tile "5" at (-1,2) with orientation 270
add tile "4" at (-1,1) with orientation 270
add tile "2" at (1,0) with orientation 90
add tile "1" at (0,1) with orientation 0
|
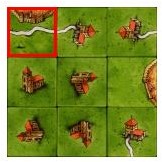 |
abbey3.ss
add tile "16" at (2,1) with orientation 90
add tile "2" at (2,2) with orientation 0
add tile "2" at (2,0) with orientation 270
add tile "2" at (1,0) with orientation 90
add tile "1" at (0,2) with orientation 270
add tile "1" at (1,2) with orientation 180
add tile "1" at (1,1) with orientation 90
add tile "1" at (0,1) with orientation 0 |
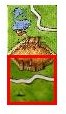 |
castle1.ss
add tile "10" at (0,-1) with orientation 180
|
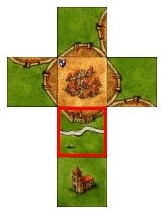 |
castle2.ss
add tile "14" at (1,-1) with orientation 180
add tile "14" at (0,-2) with orientation 90
add tile "14" at (-1,-1) with orientation 0
add tile "3" at (0,-1) with orientation 0
add tile "1" at (0,1) with orientation 0
|
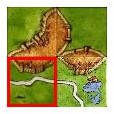 |
castle3.ss
add tile "14" at (1,-1) with orientation 180
add tile "10" at (1,0) with orientation 0
add tile "8" at (0,-1) with orientation 90
|
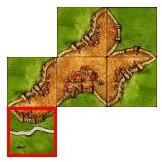 |
castle5.ss
add tile "14" at (2,-2) with orientation 180
add tile "8" at (2,-1) with orientation 270
add tile "8" at (1,-2) with orientation 90
add tile "4" at (1,-1) with orientation 0
add tile "8" at (0,-1) with orientation 90
|
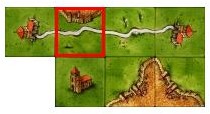 |
road0.ss
add tile "21" at (1,0) with orientation 90
add tile "2" at (2,0) with orientation 90
add tile "8" at (2,1) with orientation 180
add tile "8" at (1,1) with orientation 90
add tile "1" at (0,1) with orientation 0
add tile "2" at (-1,0) with orientation 270
|
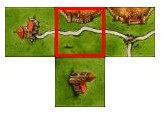 |
road1.ss
add tile "19" at (1,0) with orientation 0
add tile "2" at (-1,0) with orientation 270
add tile "1" at (0,1) with orientation 90
|
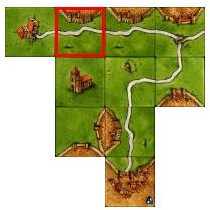 |
road2.ss
add tile "19" at (2,0) with orientation 0
add tile "17" at (2,1) with orientation 180
add tile "7" at (1,3) with orientation 180
add tile "20" at (1,2) with orientation 270
add tile "22" at (1,1) with orientation 0
add tile "20" at (1,0) with orientation 0
add tile "14" at (0,2) with orientation 90
add tile "1" at (0,1) with orientation 0
add tile "2" at (-1,0) with orientation 270
|
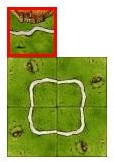 |
road3.ss
add tile "22" at (0,2) with orientation 270
add tile "22" at (1,2) with orientation 180
add tile "22" at (1,1) with orientation 90
add tile "22" at (0,1) with orientation 0
|
Task 1: Each of these graphs contains regions, some counting as
complete, some as incomplete. Turn each of these cases into a test case for
complete-region . You will naturally use insert-tile to
construct these graphs, so you will test this function implicitly. [POINTS: 6]
Task 2: Next turn each of the completed regions of these graphs into a
test case for score . [POINTS: 6]
Task 3: Finally, use the graphs to test
potential-locations-for-tile . [POINTS: 6]
In order to test the functions that find followers in a
completed region and find places where players can place followers, I have found
it convenient to develop a function that adds followers to graphs, regardless of
whether it is legal or not to place them there at the moment:
(define graph-tile<%>
(interface (tile<%>) ;; the interface for the graph
...
place-follower ;; Position Follower -> Void
;; effect: occupy the specified position on _this_ tile with the follower
;; ASSUME: the tile position is available
...
))
Using this function/method, you can modify the above graphs as follows:
image of graph | construction of graph |
|
place RED follower at (0,1) INNER
|
|
place RED follower at (0,1) INNER
|
|
place BLUE follower at (0,-1) INNER
|
|
place RED follower at (0,0) NORTH
|
|
place BLUE follower at (0,-1) INNER
place BLUE follower at (0,-2) SOUTH
|
|
place RED follower at (0,0) NORTH
place RED follower at (1,0) NORTH
place blue follower at (0,-1) INNER
|
|
place RED follower at (0,0) NORTH
place RED follower at (2,-1) INNER
|
|
place BLUE follower at (-1,0) EAST
|
|
place BLUE follower at (-1,0) EAST
place RED follower at (1,0) EAST
|
|
place BLUE follower at (-1,0) EAST
place RED follower at (1,0) EAST
place RED follower at (2,0) WEST
|
|
place BLUE follower at (0,0) EAST
|
|
place BLUE follower at (0,0) EAST
place BLUE follower at (2,0) EAST
place RED follower at (2,0) WEST
place BLACK follower at (2,0) SOUTH
|
|
place BLUE follower at (0,+1) EAST
|
Task 4: Use the first set of graphs and the above to test the function
that finds all the followers on the given region. That is, first extract the
recently completed regions, then find all the followers on these
regions. [POINTS: 6]
For potential-locations-for-followers , you can reuse the
above, making sure that your methods don't reuse positions. Also, use generate
the graphs below for additional scenarios:
|
place RED follower at (0,0) EAST
|
|
place RED follower at (0,0) EAST
|
|
place BLUE follower at (2,1) INNER
|
|
place RED follower at (1,0) NORTH
|
Task 5: Use these last two sets of graphs above to test the function
potential-locations-for-followers , which figures out where
followers can be placed. [POINTS: 6]
Product: Mail a tar bundle with your revised implementation and the
client test in a single directory. Formulate one test suite per method or
function; document the test suites and test cases so that readers know what they
test. -- Explain in a README file whether the construction of the client test
discovered any errors, and if so, what kind.
|